共计 3309 个字符,预计需要花费 9 分钟才能阅读完成。
前言
在 JavaWeb(JSP)中实现资源下载功能只需要在响应头上加入 content-disposition
响应类型即可,类型的值为attachment;filename= 文件名
,这样就可以实现下载功能。
正文
首先,我们需要一个引导页面,在引导页面中将指定的目录下的图片全部显示出来,然后输出到页面中,并提供下载按钮。代码如下:
<%@ page import="java.io.File" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Download2</title>
<style type="text/css">
a.down{
color: #FFF;
width: 150px;
height: 30px;
display: inline-block;
line-height: 30px;
background-color: #00b4ef;
text-decoration: none;
border-radius: 5px;
}
a.down:hover{opacity:0.5;}
.down_img{
border:1px dashed #ddd;
border-radius: 5px;
}
.downbox{
border:1px solid #ddd;
padding: 10px;
width: 260px;
height:160px;
box-shadow: 0 1px 3px rgba(41, 41, 41, 0.95);
display: inline-block;
margin:10px;
}
</style>
</head>
<body>
<%!
String[] checkNames = {"jpg","png","gif"}; // 可显示与下载的图片类型
public boolean check(String name){String[] s = name.split(":");
int len = s.length;
for (String names:checkNames) {if(name.equals(s[len-1])){return true;}
}
return false;
}
%>
<%
String path = request.getServletContext().getRealPath("/images");
File file = new File(path);
File[] files = file.listFiles();
for (File f:files) {if(check(f.getName())){
out.print("<div class=\"downbox\">\n" +
"<center>\n" +
"<img src=\"../images/"+f.getName()+"\"class=\"down_img\"alt=\" 加载中...\"width=\"200px\"height=\"105px\">\n" +
"</center>\n" +
"<br>\n" +
"<center><a href=\"/cn/exercise/Download2?download="+f.getName()+"\"class=\"down\"> 下载此图 </a></center>\n" +
"</div>");
}
}
%>
</body>
</html>
package cn.exercise;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet(name = "Download2", urlPatterns = {"/cn/exercise/Download2"})
public class Download2 extends HttpServlet {protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {this.doGet(request, response);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
String imgName = request.getParameter("download");
/*imgName = new String(imgName.getBytes("iso8859-1"),"utf-8");*/
String path = "/images/"+imgName;
/* System.out.println(path);*/
new Tools().fileWriter(path,response);
}
}
fileWriter
方法,这样可以实现业务逻辑分离,然后我们可以在该方法里面进行文件读入与写出,接着做出对客户端的响应。package cn.exercise;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.URLEncoder;
class Tools {void fileWriter(String path, HttpServletResponse response) throws IOException {response.setCharacterEncoding("utf-8");
path = "../../"+path;
path = this.getClass().getClassLoader().getResource(path).getPath();
File file = new File(path);
InputStream in = new FileInputStream(file);
OutputStream out = response.getOutputStream();
response.setHeader("content-disposition","attachment;filename="+ URLEncoder.encode(file.getName(),"utf-8"));
byte[] b = new byte[1024];
int length = 0;
while((length=in.read(b))!=-1){out.write(b,0,length);
}
out.close();
in.close();}
}
后记

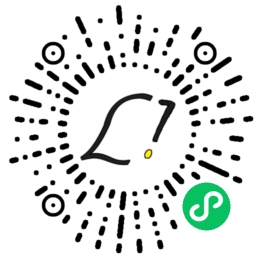